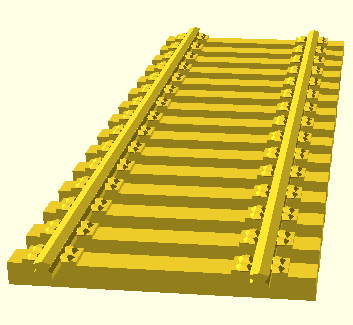
Display Train Track
This project goes with others which are in development to make a train. As of January 2018, only a preliminary version of the steam engine is done.
At this point there is also only one piece of straight track which is designed to serve as a display base for the steam engine. IF AND WHEN other parts of the train get done, maybe other kinds of track will happen.
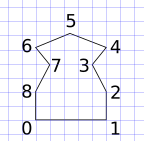
Designing the rail offered me the chance to try to do a linear extrusion of a fairly simple polygon to represent a train track. Though I did the illustration in Inkscape, the polygon was simple enough that I could figure out the points without it. The outline is sharper on top than a real rail, but it also reduces the bearing of the wheels on the track. I don't know if that will make rolling easier, if we get to that stage with the train.
Linear_extrude() serves to expand the rail polygon out to the length determined by the variable len. Using a variable here allowed easy testing of shorter track sections to verify proper rail separation for the steam engine and to see whether rail supports would be needed. Supports were not needed, but the code for support remains in case your needs vary.
The extrusion was actually the final piece of developing the track. I began with making a single tie and then put it into a module. I knew that I planned to use a for() loop to make many ties. Though the basic tie is just a cube, it also made sense to create the spikes so that they could also be included in the same loop, so I made a module to create the bracket and four spikes to put "under" the rail at each tie.
The resulting for() loop is more compact than it otherwise would be. The length of each tie also depends on the guage (distance between rails). The guage is currently 36mm between the rails which matches the steam engine's wheel separation.
// ties to support rails (count is based on length variable: len)
for(k=[0:10:len-10]){
translate([k,0,0])
tie();
// support: comment out if not needed
// translate([k-4.5,7,0])
// rotate([90,0,90])
// linear_extrude(4) support();
// translate([k-4.5,41,0])
// rotate([90,0,90])
// linear_extrude(4) support();
// end support
translate([k,-guage/2-3,4])
scale([1,1,.5])
spike();
translate([k,guage/2-3,4])
scale([1,1,.5])
spike();
}
// ties to support rails (count is based on len variable)
for(k=[0:10:len-10]){
translate([k,0,0])
tie();
}
I learned, while making the steam engine's cowcatcher, that polyhedron faces need to be defined point-by-point in clockwise order as you look from the outside of the solid you are describing. It seems that the description of a flat polygon path can be done counter-clockwise, in addition to clockwise. The polygon() command needs three things: points which identify each point on the edge of the shape by their X and Y coordinates. Polyhedron points also need a Z value because they are describing a volume, but polygons are flat, so only need values for X and Y. Then the outline needs to be described with a path which lists the points in either clockwise or counter-clockwise order. The final element of a polygon is convexity which I have only used with the default value of 10. (I guess there's more to learn, right?)
Rotating the polygon extrusion took me some experimentation, as usual. Without rotation, the rail's flat polygon extrudes straight up. These rails need to be sideways, but also upright which requires 90 degrees around the X axis and also 90 degrees around the Z axis.
Getting the rails in place involved putting each rotated rail half the guage distance out from the center of each tie, one in a positive direction along the Y axis and the other negative along the Y axis. As usual, variables are a powerful way to make projects with adjustable sizes.
module rail(){
rotate([90,0,90])
linear_extrude(len)
scale([.6,.5,.6])
polygon(points=[[0,0],[5,0],[5,2],[4,3],[5,5],[2.5,6],[0,5],[1,3],[0,2]],path=[[0,1,2,3,4,5,6,7,8,0]],convexity=10);
}
// rails
translate([-5,-guage/2,4])
rail();
translate([-5,guage/2,4])
rail();
Available Files:
These track files are the same ones found with the steam engine project. No need to download both sets.
My apologies. These files were mislabeled before 2018-04-03. The download should work now.
track01.stl - for immediate print
GPL3 License