Python Notes for Framingham Makerspace RPi
Python is a full-power programming language with relatively simple syntax rules.
It comes in two "flavors" which are different in some details.
-
The 2.7.x flavor is the current version which mixes well with projects that are in stable status.
-
The 3.2.x flavor is the future, and is recommended for projects which are just beginning and don’t depend on compatibility with some older libraries.
A simple example of the difference is the print statement:
print "This is the syntax for the 2.7 series." print("This is the syntax for the 3.2 series.")
The developers of Python wanted to bring the print command into the structure of a function. Mixing the two syntax methods will get you into trouble.
Some Basic Syntax
The traditional "Hello World" program is done like these examples.
# This is the 2.7 version. # This is a single line comment. # Any line beginning with a hash character is 'ignored' by the Python interpreter. # Commands without the beginning hash character are run as commands. print "Hello World!"
# This is the 3.2.x version. # This is a single line comment. # Any line beginning with a hash character is 'ignored' by the Python interpreter. # Commands without the beginning hash character are run as commands. print("Hello World!")
You can type the above programs using any text editor. Microsoft Word is not a text editor. Word is a more complex "What-You-See-is-What-You-Get" Word Processor. Text editors are not WYSIWYG tools. They save in the simplest text format around, ASCII (American Standard Code for Information Interchange). Choose a text editor which is intended for programming. Notepad++ is much better than the default Notepad on Windows machines, for example. The following image illustrates the code highlighting provided by the Vi(m) text editor which is built into the RPi distribution and most GNU/Linux systems. That means you can work remotely on the RPi, doing your code right there to edit your programs without needing a monitor and keyboard and mouse to get at the RPi GUI interface.
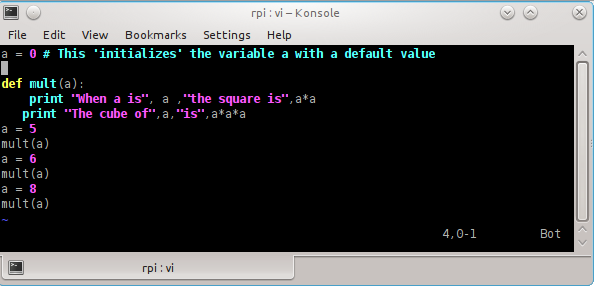
If you save this text file calling it "pyprint2.py" you can type the command below from your console and see the results. The Python interpreter defaults to version 2.7.x
python pyprint2.py Hello World!
If you wanted to do the same simple program in the newer python flavor, just swap out the print line with the 3.x version, save a new "pyprint3.py" version, and enter a slightly different command at the command prompt.
python3 pyprint3.py Hello World!
Now, learn by making a mistake. Making intentional mistakes is the best way to learn to code. Mistakes make you think about what went wrong. Seeing mistakes early and often will help you see them better when you are working on production code. Catching the "bugs" in a program is frequently more important than any other part of the job.
Try using the default Python (2.x) interpreter with the program’s version 3 file.
python pyprint3.py Hello world!
Hey, it worked! That’s because the 2.7.x interpreter has been upgraded with some of the new tricks to help legacy users.
Let’s try another intentional mistake. This one will make the program "crash" which is another term for "break."
# Intentional mistake Print "Hello World!"
Here’s what I saw on my console display.
python pyprintcap.py File "pyprintcap.py", line 3 Print "Hello world!" ^ SyntaxError: invalid syntax
The interpreter helps a little by telling us that the error is in the third line of our program. But, it shows us a caret (^) mark at the end of the line even though the mistake isn’t the quotation mark. The command "print" must be spelled just that way. It isn’t valid to say "Print" because the capital letter matters. Python commands are all lower case. Get used to seeing the tiny details. Let the interpreter help, but always recognize that you are responsible for finding the error yourself.
Strings and Variables
A string is any set of code which you intend to be repeated exactly by the Python interpreter. Text strings are conventionally enclosed between quotation marks as the word "string" here or at the beginning of the paragraph. Though it can be confusing, the quotation marks can be used so that the single quote marks are enclosing double quotes or the double quotes can enclose the single. Check this program.
# Simple string tests print "This is a simple string in double quotes." print "This is a sentence, also called a 'string'. Double quotes enclose single quotes." print 'You may use either sort of quote mark without enclosing the other sort of mark.' print 'This string uses single quotes.' print 'This is a string with the "quotes" reversed, single enclosing double.'
The output.
python strings.py This is a simple string enclosed in double-quote marks. This is a sentence, also called a 'string'. Double quotes enclose single quotes. You may use either sort of quote mark without enclosing the other sort of mark. This string uses single quotes. This is a string with the "quotes" reversed, single enclosing double.
Note
|
I hope you noticed that the program output did NOT display the quotation marks which enclosed the entire string of a sentence. However the enclosed quotes did show up. Neat. You can get quotes displayed when you need them, in this way. Later, you may also learn that you can use Python code to print a quotation mark or any other character you need without needing to use it as a string. Notice, too, that the blank lines between the print command statements do not appear in the program output in your console. They are one way to make a program easier to read or to make a block of commands more obvious. If you want a blank line, print an empty string using a command like the next. There is nothing between the two quote marks. |
print ""
Variables are a very powerful concept. With them, a programmer can assign a constant value to a "name" which makes that value easier to remember. For example, we might want to assign the current bank interest rate to a "name" of "rate". The trick is tricky because unlike strings, we do NOT use the quotation marks that have been so freely used in this paragraph. In Python, the equals sign (=) is used to make the assignment of a value to the variable name.
# Naming a value with a variable rate = 10 print rate # You can mix strings and variables in the same print statement. print "Your current interest rate is",rate,"percent." print 'Your current interest rate is', rate, 'percent.' # Note that the commas are required between the pieces of strings and the variable to help Python connect them in a series # to make a neat looking sentence for output, but that the spaces after the commas are optional, and ignored like blank lines. # Note, too that the commas cause a space, so you don't need to include space for separation of the string text from the value of the variable.
The output of this very simple use of a variable is next.
10 Your current interest rate is 10 percent. Your current interest rate is 10 percent.
You should notice that the word "rate" does not print. It is a value, not a string. The value has been assigned to the name "rate", so, instead, you get the the value of the variable displayed as the digits 10.
WAIT! Isn’t the word rate ALSO printed out as part of the string?
Good for you if you noticed that. When rate is inside the quotes, it is a string of four letters in the longer sentence. When the word rate is not enclosed in the quotation marks, it is treated as a value by Python and the current value of 10 gets printed.
Note
|
When you have a very much longer program which uses the rate value over and over, it will become clear why a single assignment of value to the variable at the beginning of the program is so wonderful. If the rate changes, you only need to change the one assignment, and the new rate will be used dozens, hundreds, or even thousands of times during the complex program. |
# Variables can also be used to store strings for reusability. # To assign a string variable value, the value must be in quotes BECAUSE it is a string. # You should also notice the convention of two-part variable names with the second part capitalized. # This is sometimes called "camelCase" because there's a hump in the middle. myName = "Algot" rate = 10 print myName," has a current interest rate of",rate,"percent."
While there is very much more to learn about using variables, this is intentionally a very limited introduction.
Functions and Indented lines
Another key concept for Python syntax formatting is strict indents. Four spaces (not a tap of the tab key) set up the inner statements of a function. No curly brackets {} or semi-colons as line terminations are required. That differentiates Python from the C-related languages.
Note
|
Actually, four spaces is not strict. You could use two all the time, or three all the time. Your programs would work, but you would be cursed the minute you tried to participate in a shared programming project. The accepted standard is four spaces for each level of indent. Any other number is going to cause an indent error. Go ahead, try using a three space indent in one of the function lines. See what happens. Never be afraid to make conscious mistakes as a learning tool. (Of course, correct the intentional mistake carefully before you move on!) |
File "func1.py", line 7 print "The cube of",a,"is",a*a*a ^ IndentationError: unindent does not match any outer indentation level
Now let’s look at a simple program with a function. Functions are groups of commands intended to be "called" at some point in a program, and to possibly be called again and again instead of being written over and over in the body of the program, making the code unnecessarily huge. (Please note, that the "over and over" shown here is not the most efficient, either. This simple document does not discuss the important concept of "loops" which make a much simpler structure for things which repeat hundreds or even thousands of times instead of only three times as this example shows.
The function begins with def, a space, and the name of the function mult(a) which needs the two parentheses and the variable which will be used by the commands in the function’s body which are the indented lines. The function definition is followed by a colon (:) to mark the beginning of the function body commands. def stands for "define." The function continues until the indented block of lines ends. It is possible to have many levels of indentation to allow for constructing complicated functions with all sorts of conditions to the commands. This simple document doesn’t cover conditionals.
# Simple function called several times # Also illustrating the concept of variable assignments. def mult(a): print("When a is ", a ,"the square is ",a*a a = 5 mult(a) a = 6 mult(a) a=8 mult(a)
The function can be expanded by adding lines that are indented the same four spaces so they are "included" in the function. Test an error on purpose again, and put the second print line in the same place, but WITHOUT the indent.
# Simple function called several times # Also illustrating the concept of variable assignments. def mult(a): print "When a is", a , "the square is", a*a print "The cube of", a ,"is", a*a*a a = 5 mult(a) a = 6 mult(a) a = 8 mult(a)
This is the error I saw.
python func1.py The cube of Traceback (most recent call last): File "func1.py", line 6, in <module> print "The cube of",a,"is",a*a*a NameError: name 'a' is not defined
This time, the error is a little more confusing to a beginner. There are actually two overlapping problems. Technically, the cube print line isn’t part of the function because it isn’t indented, but the error is actually a more fundamental one. To use a variable, it must be assigned a value, and the way we have this cube print line entered, it is before the first time we assigned a value to the variable which we called "a".
If we had indented the cube print line, all would have been fine, but since we didn’t, the Python interpreter expected us to do a preliminary assignment of value to the variable. This is usually done early, even before functions get called. So, let’s do that by adding a line above the function which assigns an initial value of zero to the variable. Do it in the currently blank line three, just below the comments.
a = 0 # This initializes the variable a with a default value
# Simple function called several times # Also illustrating the concept of variable assignments. a = 0 # This 'initializes' the variable a with a default value def mult(a): print "When a is", a ,"the square is",a*a print "The cube of",a,"is",a*a*a a = 5 mult(a) a = 6 mult(a) a = 8 mult(a)
and the new output
python func1.py The cube of 0 is 0 When a is 5 the square is 25 When a is 6 the square is 36 When a is 8 the square is 64
What you have just encountered is NOT a syntax error. There is an error, yes there is, but it is an error of programming logic. These are the more sneaky errors which don’t halt the program. The program keeps on going, and if the error is a serious one, it can lead to all the terrible stories we hear about "backdoors", "cracking", "viruses", etc.
A badly written program does what it was told to do. It just does something wrong. It won’t show up as a big deal sometimes, just like this time. There is no error in the visible output. The cube of zero is, indeed, zero. But that isn’t what we meant to do in our program. Now let’s indent the line so it is part of the function (which was our original plan) and see how the program output changes.
# Simple function called several times # Also illustrating the concept of variable assignments. a = 0 # This 'initializes' the variable a with a default value def mult(a): print "When a is", a ,"the square is",a*a print "The cube of",a,"is",a*a*a a = 5 mult(a) a = 6 mult(a) a = 8 mult(a)
And the output:
python func1.py When a is 5 the square is 25 The cube of 5 is 125 When a is 6 the square is 36 The cube of 6 is 216 When a is 8 the square is 64 The cube of 8 is 512
This time, the cubes get calculated AFTER the assignment of our intended values of 5, 6, and 8. The cube of zero is not displayed. Our program was not intended to show that line in the output. The caluclations were intended to happen when we called the function after assigning 5 and then after we assigned 6 and one last time when we called the function after assigning 8 to the variable.
This little guide just scratches the surface. You will need to do much more with Python to get the complete syntax picture, but there are tools and resources to make that easier. Some are listed below.
The IDLE Integrated Development Environment
If you are working at your RPi with a monitor, keyboard and mouse, you may prefer to use the IDLE integrated development environment (IDE). IDLE is a two-window setup which has a command interpreter in a GUI window and a separate window for a text editor. The two windows interact with one another just the way you might use Vi(m) and the regular Python interpreter remotely.
IDLE command interpreter window:
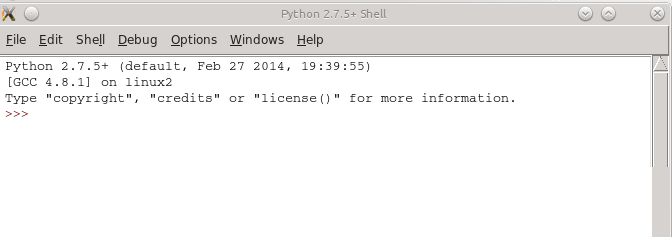
IDLE text editor window:
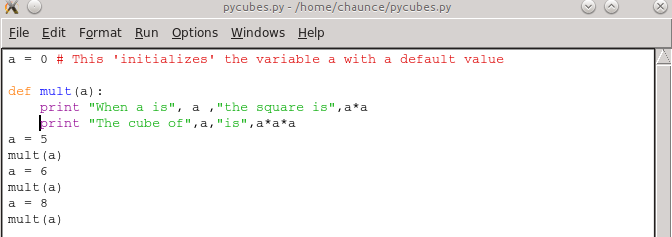
Run a program from the text editor "Run" menu or tap F5 after saving your program.
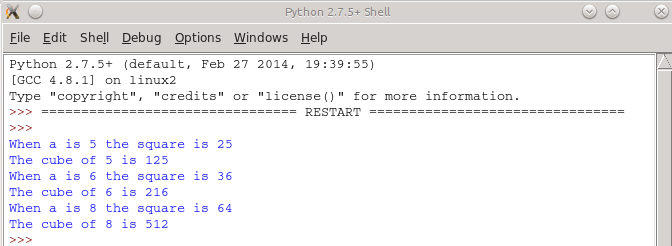
Resources
Python Online (partial list)
-
P2PU (Peer-to-Peer University) has three courses, some of which include some MIT lectures!
-
Internet Page of free book recommendations
-
I particularly like the Think Python book.
-
NOTES
Windows 7 does not come with a Python interpreter pre-installed. If you want to use Python on Windows, you’ll need to install it. One set of instructions is found at Instructables.
At the console (command line), you can also launch the python interpreter in "interactive" mode. that will allow you to test commands one at a time to see how they they work. To run Python 2.7.x just type python at the command line. You will see an introductory message and three sideways chevrons. Any python command you type will be immediately interpreted when you tap the Enter key. If you type python3 you will get the 3.2.x version installed on your computer.
Python 2.7.5+ (default, Feb 27 2014, 19:39:55) [GCC 4.8.1] on linux2 Type "help", "copyright", "credits" or "license" for more information. >>> print "Hello" Hello >>> ----- note that quitting isn't the same as the 'exit' command for the console. ----- >>> quit Use quit() or Ctrl-D (i.e. EOF) to exit >>>
The quit command is implemented as a function, for what it’s worth. Look carefully at the syntax of the command.